
What Is Assembly Language?
Assembly language is a low-level programming language that uses symbolic representations to interact with computer hardware. It is the closest language to machine language, which consists of binary code (1s and 0s), but uses mnemonic codes and symbols to make programming easier and more readable for humans. Each instruction in assembly language corresponds to a specific machine code, allowing programmers to control hardware components directly.
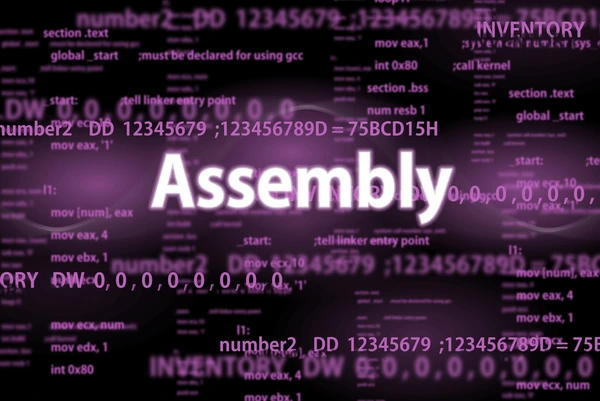
Key Characteristics of Assembly Language
- Hardware Dependency: Assembly languages are specific to a particular processor architecture, such as Intel or AMD, and even different CPUs within the same manufacturer may have unique instructions.
- Low-Level Abstraction: It provides minimal abstraction from hardware, offering detailed control over processor resources, which is useful for tasks requiring high performance or direct hardware manipulation.
- Symbolic Representation: It uses mnemonic codes and symbols to represent machine instructions and addresses, making the code more human-readable compared to machine language.
- Limited Portability: Due to its tight coupling with hardware, assembly language programs are not portable across different architectures without significant modification.
How Assembly Language Works
The underlying mechanism of assembly language operation involves translating symbolic instructions into machine code that the computer’s processor can execute directly. This translation is performed by a utility program called an assembler. Here’s a step-by-step breakdown of how it works:
- Symbolic Representation: Assembly language uses mnemonic codes and symbols to represent machine instructions. For example, the instruction
MOV
might be used to move data from one location to another. - Assembler Translation: The assembler takes the assembly code as input and generates machine code as output. It translates mnemonic codes and symbolic representations into the corresponding machine code instructions and binary values.
- Machine Code Execution: The generated machine code is executed by the computer’s processor. Since assembly language is closer to machine code, the programs written in assembly language can run faster and be more efficient in terms of size compared to high-level languages.
Structure of Assembly Language
The structure of assembly language is closely related to its underlying mechanism of operation. The symbolic representation and mnemonic codes used in assembly language are designed to reflect the machine instructions and operations defined by the processor’s ISA. This allows programmers to write code that is easily translated into machine code, facilitating direct control over the hardware. The structure enables efficient execution of instructions, but also requires a good understanding of the processor’s architecture and instruction set, which can be a barrier to learning and use.
Advantages of Assembly Language
- High Control and Efficiency: Assembly language offers direct control over hardware resources, leading to potentially faster and more efficient programs.
- Portability: Assembly language programs can be relatively portable across different CPU architectures with minimal modifications.
- Low-Level Access: It provides easy access to hardware components, which is useful for tasks requiring precise control, such as device drivers and embedded systems.
Disadvantages of Assembly Language
- Error-Prone and Time-Consuming: Writing assembly code can be error-prone and time-consuming due to the need for manual management of memory and registers.
- Non-Intuitive and Less Productive: Assembly language is often less intuitive and less productive than high-level languages, requiring a deeper understanding of the computer’s hardware.
- Maintenance Challenges: Programs written in assembly language can be difficult to maintain and modify due to their low-level nature and the need for precise code changes.
- Development Speed: Development speed is generally lower in assembly language compared to high-level languages, which provide more abstractions and automation.
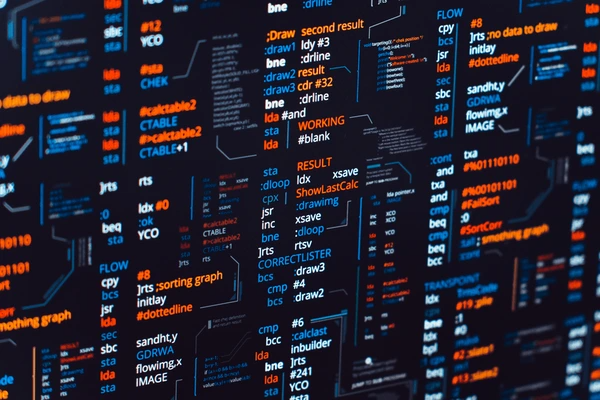
Assembly Language vs. High-Level Languages
Level of Abstraction
- Assembly Language: Much closer to machine code, with a one-to-one correspondence between assembly instructions and machine code instructions. It uses mnemonic codes to represent machine instructions, making it understandable by humans.
- High-Level Languages: Abstracts away many low-level details, using syntax and concepts that are closer to human reasoning. It provides higher-level constructs and abstractions, allowing programmers to focus on problem-solving rather than hardware details.
Syntax and Constructs
- Assembly Language: Syntax is processor-specific and closely tied to the architecture of the computer. Instructions are typically short and directly map to hardware operations.
- High-Level Languages: Syntax is more human-readable and portable across different architectures. It uses constructs like loops, conditionals, and data types that are easier to understand and maintain.
Interaction with Hardware
- Assembly Language: Provides direct access to hardware components such as registers and memory. It allows fine-grained control over hardware operations, which can be crucial for performance-critical applications.
- High-Level Languages: Abstracts the hardware details, providing a layer of indirection through the operating system. This makes it easier to write portable code but can introduce performance overhead.
Compilation and Execution
- Assembly Language: Typically assembled into machine code using an assembler, which translates the mnemonic codes into binary machine code. It can be executed directly by the CPU.
- High-Level Languages: Compiled into machine code by a compiler, which generates multiple versions for different architectures. The resulting machine code is executed by the CPU.
Development and Maintenance
- Assembly Language: Requires a deeper understanding of the computer architecture and can be more error-prone due to the need for manual memory and register management. Code is often less portable across different systems.
- High-Level Languages: Easier to develop and maintain due to higher-level abstractions and better error-handling mechanisms. Code is generally more portable and easier to read and modify.
Common Assemblers and Tools
- Assemblers: MASM (Microsoft Macro Assembler), NASM (Netwide Assembler), and GAS (GNU Assembler).
- Debuggers: OllyDbg, IDA Pro, and GDB (GNU Debugger).
- Simulators: Visual Simulator for Assembly Language Programming, which provides a visual simulation of machine and assembly language programs.
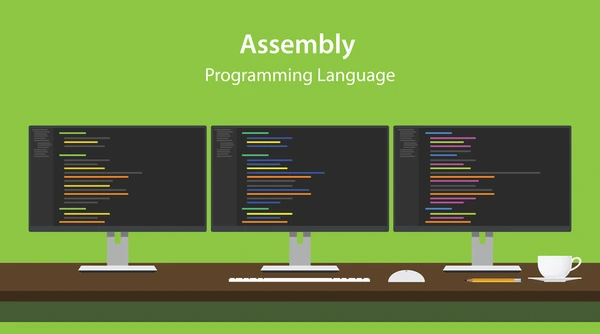
Applications of Assembly Language
Embedded Systems and Microprocessors
- Assembly language is predominantly used in embedded microprocessors where performance and efficiency are critical. It allows for the development of programs that are faster and more compact compared to high-level languages, providing greater control over the program applications.
Optimization and Performance-Critical Applications
- In scenarios where further optimization is needed beyond what compilers can achieve, assembly language is employed. This is particularly true for applications with limitations on execution speed or program size, such as in kernel and device driver development.
Low-Level System Programming
- The need to control CPU operations directly makes assembly language indispensable for low-level system programming. It is used when fine-grained control over hardware resources like memory and arithmetic execution speed is required.
Mixed-Language Programming
- Inline assembly is used within high-level language programs to optimize performance-critical sections. This allows developers to include assembly language descriptions directly in high-level language programs, achieving execution speeds close to those of programs written entirely in assembly language.
Legacy System Maintenance and Development
- Assembly language is still used in maintaining and developing legacy systems where existing codebase is in assembly, and transitioning to a higher-level language might not be feasible due to complexity or performance concerns.
Security-Critical Applications
- Due to its low-level nature, assembly language is sometimes used in security-critical applications where understanding and controlling the exact behavior of the code is essential.
Latest Technical Innovations in Assembly Language
- Embedded Systems: Assembly language is widely used in embedded systems due to its efficiency and low-level control capabilities. It is particularly useful for programming microcontrollers and other small-scale devices where performance and resource constraints are critical.
- Real-Time Systems: In real-time systems, where precise timing is crucial, assembly language is often employed to ensure that tasks are completed within strict deadlines. Its ability to directly manipulate hardware resources makes it ideal for such applications.
- High-Performance Computing: Assembly language is used in high-performance computing applications, such as scientific simulations and data processing, where every cycle counts. It allows developers to optimize code for specific hardware architectures, leading to significant performance gains.
- Firmware Development: Firmware for various devices, including IoT devices, is often written in assembly language. This is because firmware needs to be highly efficient and reliable, and assembly language provides the necessary control and performance.
- Security Applications: Assembly language is used in security applications, such as malware development and reverse engineering, due to its low-level nature and ability to bypass high-level language security measures. However, it is also used in creating secure firmware and software components.
- Low-Level System Programming: Assembly language is used for low-level system programming tasks, such as device drivers and operating system kernels. Its direct access to hardware resources makes it suitable for such tasks.
- Specialized Hardware: Assembly language is used in programming specialized hardware, such as FPGAs (Field-Programmable Gate Arrays) and ASICs (Application-Specific Integrated Circuits). It allows developers to optimize code for specific hardware configurations, leading to improved performance and efficiency.
FAQs
- What is the difference between assembly language and machine code?
Assembly language uses human-readable mnemonics, while machine code consists of binary instructions directly executed by the CPU. - Why is assembly language still used today?
It’s used for performance-critical tasks, embedded systems, and scenarios requiring direct hardware interaction. - Can assembly language be learned without prior programming experience?
While possible, prior knowledge of basic programming concepts can make learning assembly easier. - How does assembly language handle memory management?
Assembly provides explicit control over memory through instructions likeLOAD
,STORE
, and memory address manipulation. - What are the alternatives to assembly language for low-level programming?
C and C++ offer low-level capabilities with better abstraction and portability.
To get detailed scientific explanations of assembly language, try Patsnap Eureka.
